While in Part 1 we saw how could we set up the project, and get a cron running to pull the products from the database and show the products on the UI.
Here is a sneak peak of the same.
Here in this post, how about analyzing the stocks column of the product details in the database, and using this count to decide sending a email to the owner that the stock is going to be replenished and he is supposed to add more numbers of stocks.
If we analyze the run_task_cron_def class method, here are the couple of stuffs we are doing.
class ProductsCron():
def __init__(self, list_of_products, json_object=None):
self.list_of_products = list_of_products
self.json_object = json_object
def runCron(self, type):
self.list_of_products = serializers.serialize(
'json', ProductsManager().get_queryset())
print("Cron Is Running At Every 1 minute")
return HttpResponse(self.list_of_products, content_type="application/json")
def run_task_cron_def(self, isOutOfStock):
inventory_products = serializers.serialize(
'json', ProductsManager().get_queryset())
# convert string to object
self.json_object = json.loads(inventory_products)
if (len(self.json_object) > 0):
for x in range(len(self.json_object)):
if (self.json_object[x]["fields"]["stocks"] <= '10'):
stock_status = serializers.serialize(
'json', ProductsManager().get_stocks(str(self.json_object[x]["fields"]["stocks"])))
stocks = str(json.loads(stock_status)
[x]["fields"]["stocks"])
title = str(json.loads(stock_status)[x]["fields"]["title"])
price = str(json.loads(stock_status)[x]["fields"]["price"])
self.sendEmail(stocks, title, price)
print("run_task_cron_def Cron Is Running At Every 1 minute")
def sendEmail(self, stocks, title, price):
text = f'This Product - {title} of Price {price} is left only {stocks}..'
from_email = 'pallavidapriya75@gmail.com'
to_emails = ['priyaarshinipallavi@gmail.com',
'htfhfhfhfh#gamil.com', 'writerpro2025@gmail.com']
emails_valid_invalid = self.validate_email(to_emails)
print("Successfully did not sent email message to %s: It is invalid mail" %
(", ".join(emails_valid_invalid[1])))
subject = 'Inventory Goods Update'
assert isinstance(emails_valid_invalid[0], list)
msg = MIMEMultipart('alternative')
msg['From'] = from_email
msg['To'] = ", ".join(emails_valid_invalid[0])
msg['Subject'] = subject
txt_part = MIMEText(text, 'plain')
msg.attach(txt_part)
html_part = MIMEText(
"This Product - {0} of Price {1} is left only {2}..".format(title, price, stocks))
msg.attach(html_part)
msg_str = msg.as_string()
self.send_mail(from_email, emails_valid_invalid[0], msg_str)
def validate_email(self, emails):
valid_emails = []
invalid_emails = []
for email in emails:
if re.match(r"[^@]+@[^@]+\.[^@]+", email):
valid_emails.append(email)
else:
invalid_emails.append(email)
return valid_emails, invalid_emails
def send_mail(self, from_email, to_emails, msg_str):
username = 'pallavidapriya75@gmail.com'
password = 'xvrx dkbu tsqs ybkq'
server = smtplib.SMTP(host='smtp.gmail.com', port=587)
server.ehlo()
server.starttls()
server.login(username, password)
print("Email Successfully sent to %s: For a product inventory update" %
", ".join(to_emails))
for email in to_emails:
server.sendmail(
from_email, email, msg_str)
We are getting all the product information in that and converting to json object and then passing the stock to an other method where we pull the following data from the table- id, price, title, stocks.
def get_stocks(self, num):
string_stock = str(num)
return Products.objects.raw("SELECT id, price, title, stocks FROM public.products_products WHERE stocks = %s", [string_stock])
Finally sending all the data to another class method sendMail where we format the message to be sent to the owner along with the email validation part.
Finally, we are executing send_mail method where are using smtp to send the actual email.
Here is a sneak peak of the server logs and email we get respectively.

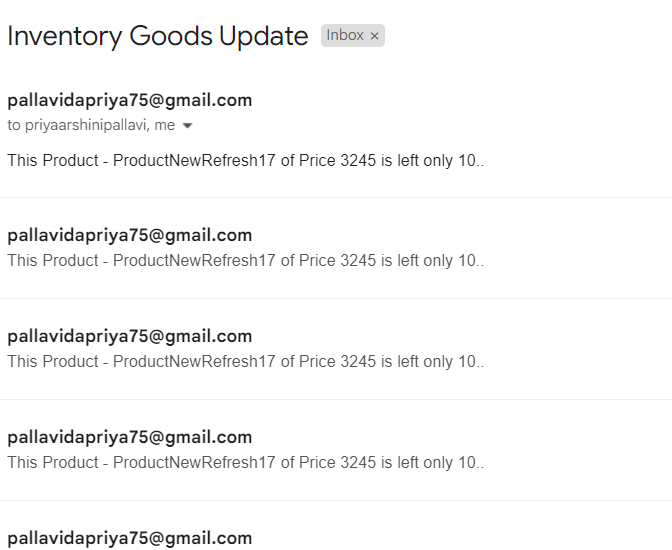
That is it for this where we send emails, but this series has a part3 as well. so let’s check what is it.