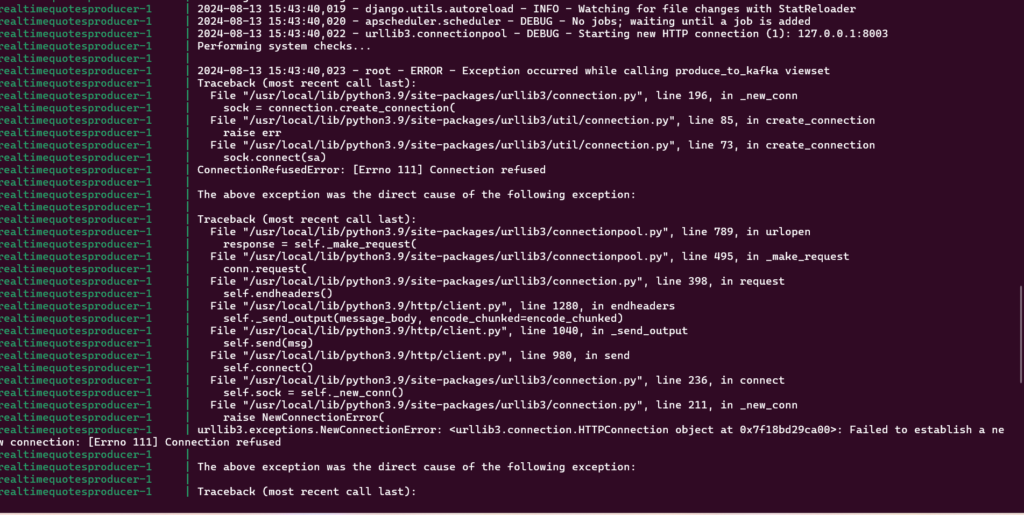
Do you see the logs? Do you like seeing it like this? No right? Who dont like clean logs. I do. But this was the original flow which was causing the problem.
I have a scheduler which runs kafka producer that is called when the app is initialized, but the problem was it was getting started before the server itself started.
This was the initial flow:
Original Flow (Problematic)
- Django Starts Initialization:
- The Django application begins to initialize, calling the
ready()
method of each app.
- The Django application begins to initialize, calling the
- Scheduler Starts Early:
- The scheduler is started in the
ready()
method, before the Django server is fully up and ready to handle requests.
- The scheduler is started in the
- Server Not Ready:
- The scheduler may try to add jobs that involve interacting with the Django server, but since the server isn’t ready yet, this leads to connection errors or the scheduler shutting down prematurely.
Why the Scheduler Started First
- Immediate Execution: When the scheduler was originally started within the
ready()
method, it was initiated as soon as Django began initializing the app. This means the scheduler was started before the server was fully up and running, because the server process (that listens for incoming requests) is typically not ready until after the entire initialization process is complete. - No Delay: Without any delay or checks for server readiness, the scheduler would try to connect to the Django server or perform tasks that required the server to be fully operational, leading to issues if the server wasn’t ready.
Revised Flow (Corrected)
- Django Starts Initialization:
- The Django application begins to initialize, but now the scheduler is not started immediately.
- Server Fully Starts:
- The Django server completes its startup process and begins accepting connections.
- Scheduler Starts After Delay:
- The scheduler starts after a delay, ensuring the server is fully ready. The delay allows the main process (Django server) to finish its startup sequence.
- Jobs Added to Scheduler:
- The scheduler adds jobs and begins executing them, with the assurance that the server is now ready to handle any requests or tasks those jobs might involve.
What techniques I used to get this correct?
1. Context Managers
- Definition: Context managers are used to manage the setup and teardown of resources in a clean and reusable way. In Python, they are implemented using the
with
statement and thecontextlib
module. - In the Code:
managed_scheduler
andwait_for_django_server
are both context managers. They ensure that the scheduler is started and stopped cleanly and that the server is ready before proceeding.
def start_scheduler():
server_url = 'http://127.0.0.1:8003/quotes_producer/stockdata/producetokafka/' # Adjust as needed
with wait_for_django_server(server_url) as server_ready:
if server_ready:
# Start the scheduler and add the job
with managed_scheduler() as scheduler:
try:
# Add the job to the scheduler
logger.info("Attempting to add job to scheduler.")
trigger = DateTrigger(run_date=None) # `None` means "now"
scheduler.add_job(call_produce_to_kafka_viewset, trigger=trigger)
logger.info("Job added to scheduler.")
except Exception as e:
logger.error(f"Failed to add job to scheduler: {e}")
# Keep the scheduler running
logger.info("Scheduler is now waiting for jobs.")
try:
while True: # Keep the scheduler alive
time.sleep(1)
except (KeyboardInterrupt, SystemExit):
logger.info("Scheduler stopped manually.")
else:
logger.error("Failed to start scheduler as the Django server is not ready.")
2. Threading
- Definition: Threading allows multiple threads (smaller units of a process) to run concurrently within a single process. This can be useful for performing background tasks without blocking the main execution flow.
- In the Code:
- The
ready
method in theCommand
class starts a new thread (Thread(target=start_scheduler_thread)
) to run the scheduler. This allows the Django server to complete its startup process while the scheduler is initialized in parallel.
- The
def ready(self):
if os.environ.get('RUN_MAIN') == 'true': # Ensure this runs only in the main process
def start_scheduler_thread():
time.sleep(10) # Allow Django to fully start up
from django.core.management import call_command
call_command('runscheduler')
# Start the scheduler in a new thread
thread = Thread(target=start_scheduler_thread)
thread.start()
3. Retry Logic with Exponential Backoff
- Definition: Retry logic involves repeatedly attempting an operation that might fail, with some delay between attempts. Exponential backoff is a strategy where the delay between retries increases exponentially, which helps in scenarios like network connections where a service might take time to become available.
- In the Code:
- The
wait_for_django_server
context manager implements retry logic with exponential backoff. It tries to connect to the server at increasing intervals until the server becomes available or a timeout occurs.
- The
@contextmanager
def wait_for_django_server(url, timeout=120, initial_retry_interval=1):
"""Context manager to wait until the Django server at `url` is available or timeout occurs."""
start_time = time.time()
retry_interval = initial_retry_interval
while time.time() - start_time < timeout:
try:
response = requests.post(url)
if response.status_code == 200:
print("Django server is up and running.")
yield True # Server is ready, proceed with scheduler
return
except ConnectionError:
print(f"Django server is not ready yet, retrying in {retry_interval} seconds...")
time.sleep(retry_interval) # Wait before retrying
retry_interval = min(retry_interval * 2, 60) # Exponential backoff with a max wait time of 60 seconds
print("Django server did not start in time.")
yield False # Server did not start in time
4. Logging
- Definition: Logging is the practice of recording information about a program’s execution, which can be crucial for debugging and monitoring.
- In the Code:
- The
logger
is used throughout the code to record important events, such as when the scheduler starts and stops, when a job is added, or when errors occur. This makes it easier to diagnose problems.
- The
5. Django Management Commands
- Definition: Django management commands are custom commands that can be run using Django’s
manage.py
. They allow developers to extend Django’s command-line interface. - In the Code:
- The
Command
class defines a custom Django management command that starts the scheduler. Thehandle
method is the entry point when the command is executed.
- The
6. Conditionals
- Definition: Conditional statements (
if
,else
) allow the program to execute different code paths based on certain conditions. - In the Code:
- Conditionals are used to check if the server is ready, to decide whether to start the scheduler, and to manage the flow of the program (e.g.,
if server_ready:
).
- Conditionals are used to check if the server is ready, to decide whether to start the scheduler, and to manage the flow of the program (e.g.,
7. Resource Management
- Definition: Resource management involves ensuring that resources like memory, file handles, or network connections are properly allocated and released.
- In the Code:
- The context managers (
managed_scheduler
,wait_for_django_server
) handle the allocation and release of resources. For example,managed_scheduler
ensures that the scheduler is started and cleanly shut down, even if an error occurs.
- The context managers (
8. Python’s atexit
Module
- Definition: The
atexit
module allows you to register functions to be called when a program is terminating. This is useful for cleanup operations. - In the Code:
- The
stop_scheduler
function is registered withatexit.register(stop_scheduler)
, ensuring that the scheduler is properly shut down when the application exits.
- The
9. Exception Handling
- Definition: Exception handling involves catching and managing errors that occur during program execution, allowing the program to fail gracefully or attempt recovery.
- In the Code:
try-except
blocks are used to catch exceptions, such as connection errors or issues when adding jobs to the scheduler. This prevents the program from crashing and allows for logging the error or attempting recovery.
10. Python’s time
Module
- Definition: The
time
module provides various time-related functions, such as sleeping for a certain period or measuring time intervals. - In the Code:
time.sleep()
is used in thewait_for_django_server
function to implement the retry logic, and in theready
method to delay the start of the scheduler until after the Django server has had time to initialize.
These concepts work together to create a robust, fault-tolerant system where a background task (the scheduler) is started and managed independently of the main application flow, ensuring that it runs only when the server is fully ready and continues to run smoothly without interruptions.